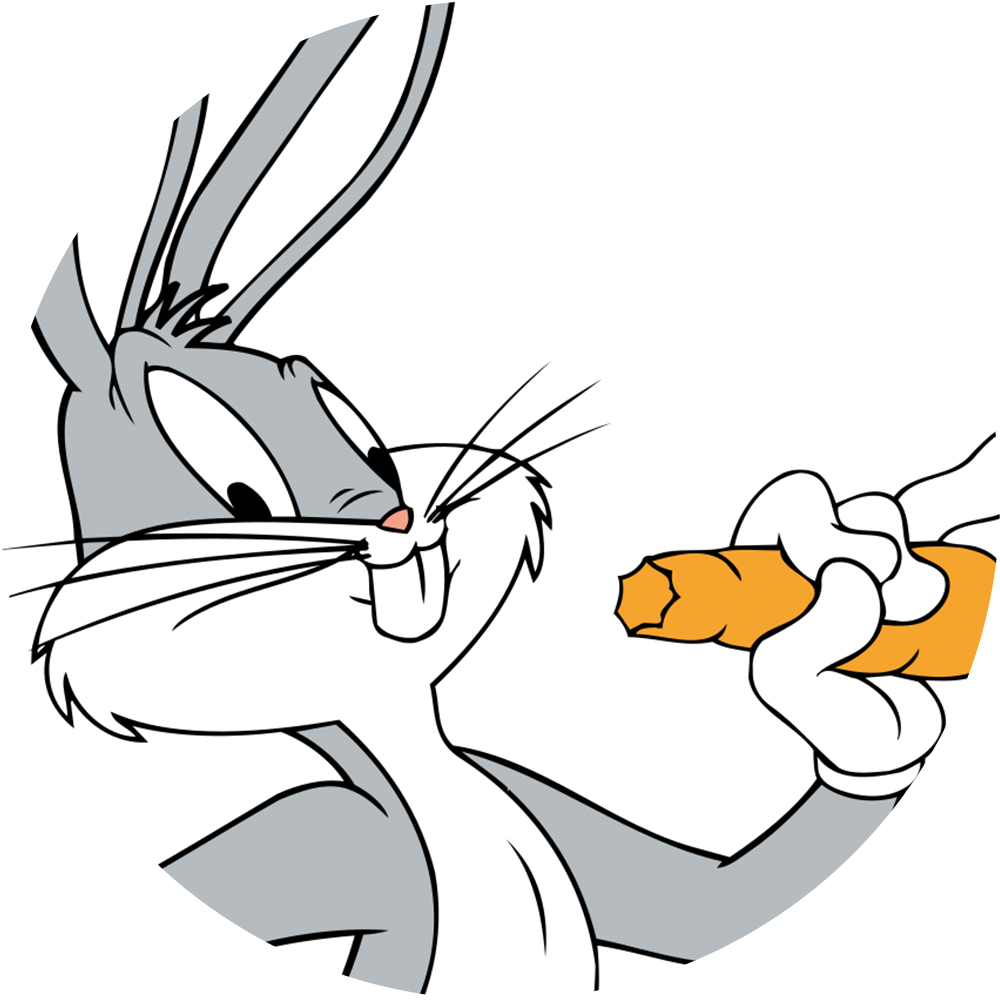
The power of PHP annotations
I was quite intrigued when I learned a while back that PHP documentation comments are available via reflection. This is the first language where I’ve seen this. This means that given the following code:
class AnyClass {
/**
* @param string $s
*/
public function anyFunc($s) {
// Any code
}
}
You can actually get a hold of the comment block above anyFunc
from your PHP script:
function getComment() {
$c = new ReflectionClass('AnyClass');
$m = $c->getMethod('anyFunc');
return $m->getDocComment();
}
With a little parsing effort this effectively gives PHP what Java calls annotations and C# calls Attributes – A means to add meta data to your code that can be used in your logic. Not sure PHP have a name for this, but I’ll call it PHP annotations.
function getAnnotations() {
$c = new ReflectionClass('AnyClass');
$m = $c->getMethod('anyFunc');
$s = $m->getDocComment();
$s = str_replace('/*', '', $s);
$s = str_replace('*/', '', $s);
$s = str_replace('*', '', $s);
$aTags = explode('@', $s);
return $aTags;
}
From this I was able to build a small little MVC framework that power-use these PHP annotations. For example here is a MVC controller action function:
/**
* @param code is required
* @param name is required
* @param query is required
* @loadview stored-query-save
* @ajaxify
*/
public function storedQuerySaveAction() {
// At this point the framework made
// sure the HTTP request contains
// something in the code, name and
// query variables
// When this action function
// returns the framework will
// render a view called stored-query-save
// and the @ajaxify annotation will
// cause the framework to add
// some jQuery magic to the
// output to provide a better user
// experience through the use
// of ajax techniques.
}